How I would learn programming from scratch
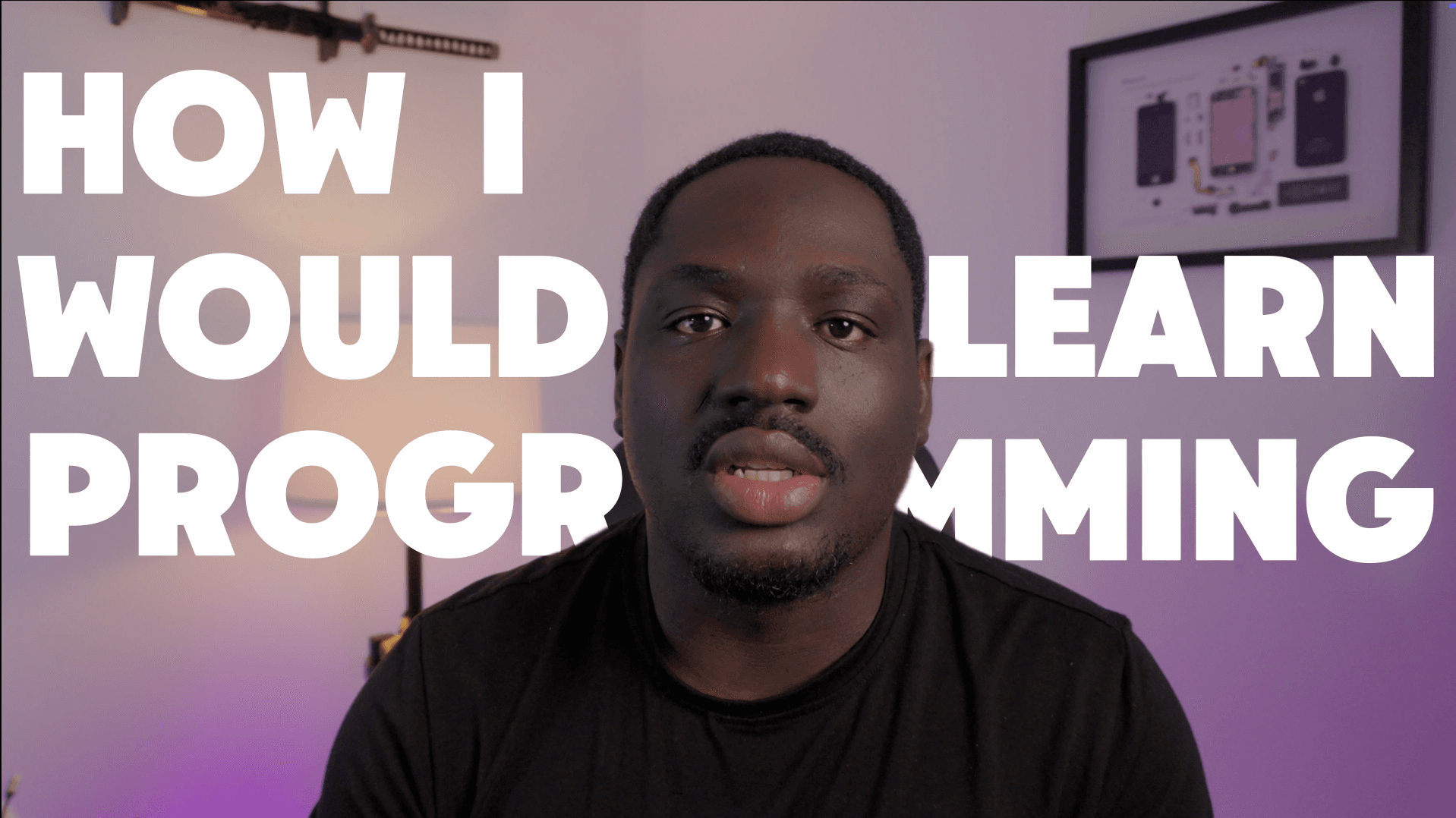
Here are 5 things I'd do if I were learning programming from scratch.
1. learn HTML & CSS
HTML (HyperText Markup Language) is the foundation of every web page. It’s a markup language used to structure content on the web. Think of it as the skeleton of a website—it tells the browser what each part of the webpage is and how it should be organized. Learning HTML & CSS gives you the foundation to build web apps
Resources
- W3Schools: Learn HTML
- W3Schools: Learn CSS
- Youtube: HTML Full Course for Beginners
- Youtube: CSS Full Course for Beginners
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>My Website</title>
<link rel="stylesheet" href="./style.css">
<link rel="icon" href="./favicon.ico" type="image/x-icon">
</head>
<body>
<main>
<h1>Welcome to My Website</h1>
</main>
<script src="index.js"></script>
</body>
</html>
2. Learn Python
Python, a simple and easy-to-understand programming language, requires minimal setup and has many applications. It excels in web development, data science, automation, game development, and artificial intelligence. The extensive library ecosystem and active community provide solutions to complex tasks and learning resources. Its popularity across industries and adaptability make it suitable for both beginners and experienced developers.
Resources
- W3Schools: Learn Python
- Youtube: Learn Python | Full 7-Hour Course
- Youtube: Python Full Course for Beginners
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def myfunc(self):
print("Hello my name is " + self.name)
p1 = Person("John", 36)
p1.myfunc()
3. Learn Databases
Databases are organized systems for storing, managing, and retrieving data. They enable applications to handle vast amounts of information efficiently, making them essential for websites, apps, and businesses. Common types include relational databases (e.g., MySQL, PostgreSQL) and non-relational (NoSQL) databases (e.g., MongoDB).
SELECT * FROM Users where active = 1;
SQL (Structured Query Language) is the standard language for interacting with relational databases. It allows you to:
- Create databases and tables.
- Insert new data.
- Retrieve data with queries (e.g., SELECT * FROM users;).
- Update existing records.
- Delete data as needed.
- Learning SQL is crucial because it simplifies working with structured data, enabling developers to build dynamic, data-driven applications like e-commerce sites, social networks, and more.
Resources
- Databases and SQL
- Intro to Relational Databases
- Youtube: SQL Tutorial for Beginners
- Youtube: SQL Course for Beginners
4. Learn Javascript
JavaScript is a powerful, versatile programming language primarily used to create dynamic and interactive web content. It allows developers to add features like animations, form validations, dropdown menus, and real-time updates to websites. JavaScript works alongside HTML and CSS to bring web pages to life, making it a cornerstone of web development.
Beyond the browser, JavaScript is also used on servers (via Node.js), in mobile app development (React Native), and even for game development. Its flexibility, extensive ecosystem of libraries and frameworks, and ease of integration make it one of the most popular programming languages in the world. Whether you're building a simple website or a complex application, JavaScript is an essential tool for modern developers.
Resources
- W3Schools: Learn Javascript
- The Modern JavaScript Tutorial
- Build 30 things in 30 days with 30 tutorials
- Youtube: JavaScript Crash Course For Beginners
- Youtube: Build a Netflix Landing Page Clone with HTML, CSS & JS
function toCelsius(f) {
return (5/9) * (f-32);
}
let value = toCelsius(77);
console.log('Value in Celsius', value)
5. Build Projects
Building projects is a crucial step in learning programming, as it bridges the gap between theory and practical application. Projects allow you to apply your knowledge, solve real-world problems, and develop a deeper understanding of programming concepts. They also enhance your problem-solving skills and boost creativity by challenging you to think critically.
Starting with small projects, like a calculator or a to-do list app, helps solidify foundational skills. Gradually, you can progress to more complex tasks, such as creating a portfolio website, an e-commerce platform, or even a game. Each project becomes a showcase of your abilities, providing tangible proof of your skills to potential employers or collaborators. Building projects isn't just practice—it's the key to mastering programming and standing out in the tech world.